How to check if image was upscaled
Detecting low-quality images programatiacally
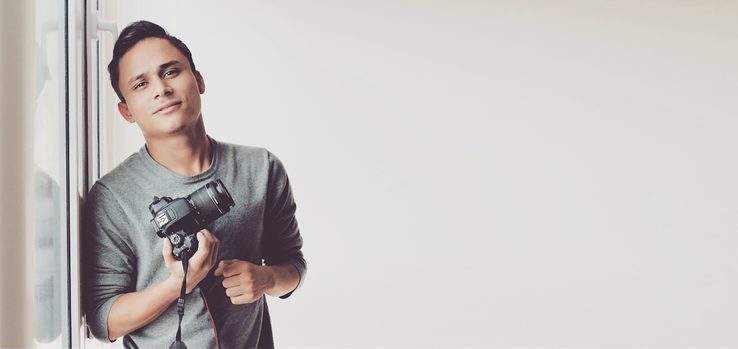
<?php
$url = 'http://your_server.com/image.png';
$data = json_decode(file_get_contents('http://api.rest7.com/v1/image_upscaled.php?url=' . $url));
if (@$data->success !== 1)
{
die('Failed');
}
if ($data->is_upscaled)
{
echo 'The image was upscaled. It was originally ' . $data->original_width . 'x' . $data->original_height;
}
So how does this work?
Let's say we have this image, 300x200:
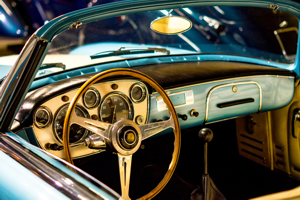
Now we upscale it to 600x400 using Lanczos:
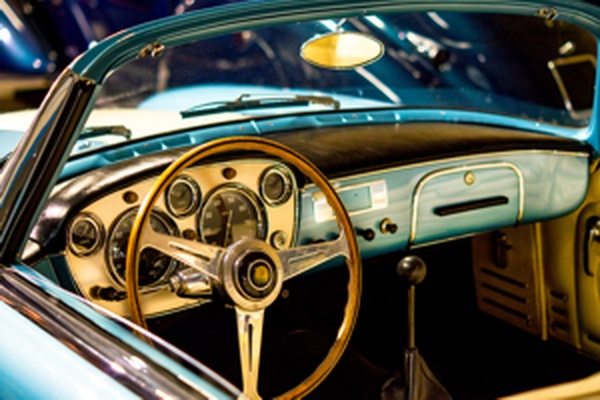
{
"is_upscaled": true,
"current_width": "600",
"current_height": "400",
"original_width": 300,
"original_height": 200,
"accuracy": "68%",
"accuracy_width": "67%",
"accuracy_height": "69%",
"cropped": "",
"success": 1
}
Then this array is parsed by our script and it prints:The image was upscaled. It was originally 300x200
Comments